- Download a Precompiled DLL of SQLite http://www.sqlite.org/download.html
- Move the sqlite3.dll and sqlite3.def to Assets/Plugins in your unity project
- Download SQLite Browser http://sourceforge.net/projects/sqlitebrowser/ or using SQLite Manager addons of Mozilla Firefox.
- Create a database in your Assets/ folder in your unity project with SQLite broswer
- Copy System.Data.dll and Mono.Data.Sqlite.dll from C:\Program Files (x86)\Unity\Editor\Data\Mono\lib\mono\2.0\ and paste them in your Assets/ folder in your unity project.
- Access the database as above, only where “/GameMaster” is put “/YourDbName”. Note if you did this correctly MonoDevelop should have all of the methods when you type reader.
- When building you must copy your database file to the folder that automatically is created called yourProject_Data wherever you saved the executable.
Script:
using UnityEngine; using System.Collections; using Mono.Data.Sqlite; using System.Data; using System; public class SqliteDb : MonoBehaviour { // Use this for initialization void Start () { string connectionString = "URI=file:" +Application.dataPath + "/sampledb.sqlite"; //Path to database. IDbConnection dbcon; dbcon = (IDbConnection) new SqliteConnection(connectionString); dbcon.Open(); //Open connection to the database. IDbCommand dbcmd = dbcon.CreateCommand(); string sql = "SELECT col1, col2 FROM `sampletable`"; dbcmd.CommandText = sql; IDataReader reader = dbcmd.ExecuteReader(); while(reader.Read()) { int Col1 = reader.GetInt16 (0); string Col2 = reader.GetString (1); Console.WriteLine(Col1 + " " + Col2); Debug.Log (Col1 + Col2); } // clean up reader.Close(); reader = null; dbcmd.Dispose(); dbcmd = null; dbcon.Close(); dbcon = null; } // Update is called once per frame void Update () { } }
Unity console.
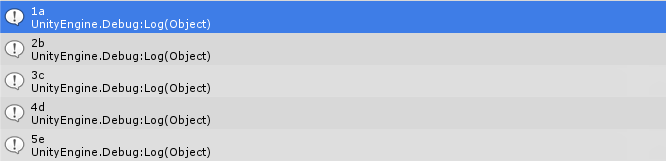
Important Copy sqlite3.dll into your into your project's Plugins folder (make a folder called Plugins if you don't have one).
- You won't get a warning if you don't do this, and your project will run fine in the editor, however, it will fail to work when you actually build your project, and will only provide information about this in the log file.
- This will give you a “License error. This plugin is only supported in Unity Pro!” if you're using Unity Indie, but it doesn't seem to have an effect on the actual play in the editor, nor does it seem to effect the ability to build stand-alone versions.
- Alternately, you can leave it out of your project entirely, but when you build your application, you'll need to include a copy of sqlite3.dll in the same directory as the .exe in order for it to work.
No comments:
Post a Comment
Silahkan